728x90
SMALL
package com.jojoldu.book.springboot.domain.posts;
import org.junit.After;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.test.context.junit4.SpringRunner;
import java.util.List;
import static org.assertj.core.api.Assertions.assertThat;
@RunWith(SpringRunner.class)
@SpringBootTest // 별다른 설정이 없을 경우 H2 데이터베이스를 자동으로 실행.
public class PostsRepositoryTest {
@Autowired
PostsRepository postsRepository;
@After // Junit에서 단위 테스트가 끝날 때마다 수행되는 메소드를 지정.
public void cleanup(){
postsRepository.deleteAll();
}
@Test
public void 게시글저장_불러오기(){
// given
String title = "테스트 게시글";
String content = "테스트 본문";
postsRepository.save(Posts.builder() // 테이블 posts에 insert/update 쿼리를 실행. id 값이 있다면 update가, 없다면 insert 쿼리가 실행.
.title(title)
.content(content)
.author("jadejung95@gmail.com")
.build());
//when
List<Posts> postsList = postsRepository.findAll(); // 테이블 posts에 있는 모든 데이터를 조회해오는 메소드
//then
Posts posts = postsList.get(0);
assertThat(posts.getTitle()).isEqualTo(title);
assertThat(posts.getContent()).isEqualTo(content);
}
}
실제로 실행된 쿼리는 어떤 형태인 지 로그로 확인하려면 ?
: 스프링 부트에서는 application.properties, application.yml 등의 파일로 한 줄의 코드로 설정할 수 있도록 지원하고 권장한다.
src/main/resource 디렉토리 아래에 application.properties 파일을 생성한 후
spring.jpa.show_sql=true 옵션을 추가한다.
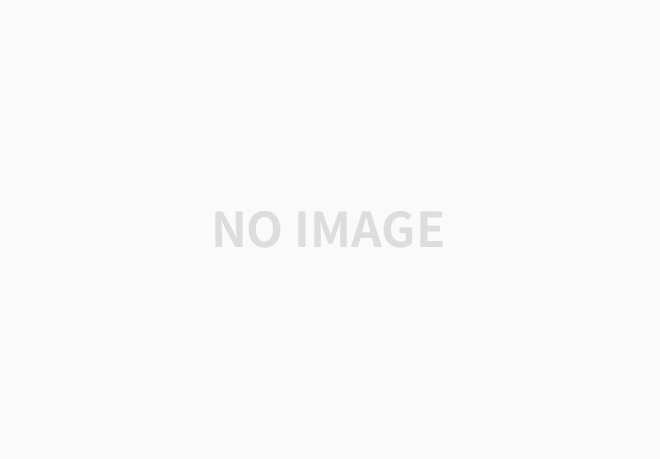
그리고 테스트를 실행하면 콘솔에서 쿼리 로그를 확인할 수 있다.
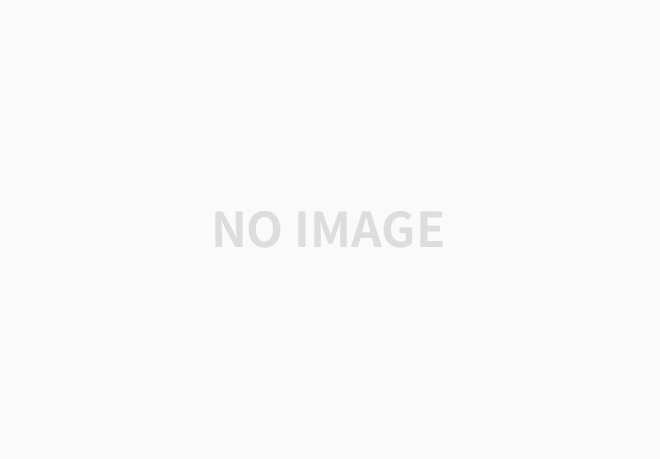
다만 , create table 쿼리를 보면 id bigint generated by default as identity 라는 옵션으로 생성된다.
이는 H2 쿼리의 문법이 적용되었기 때문이다.
출력되는 쿼리 로그를 MySQL 버전으로 변경해보자. (H2는 MySQL의 쿼리를 수행해도 정상적으로 작동한다)
application.properties 파일에 다음 코드를 추가하면 된다.
spring.jpa.properties.hibernate.dialect=org.hibernate.dialect.MySQL5InnoDBDialect
추가한 후 다시 테스트를 실행해보면. 다음과 같이 옵션이 잘 적용되었음을 확인할 수 있다.
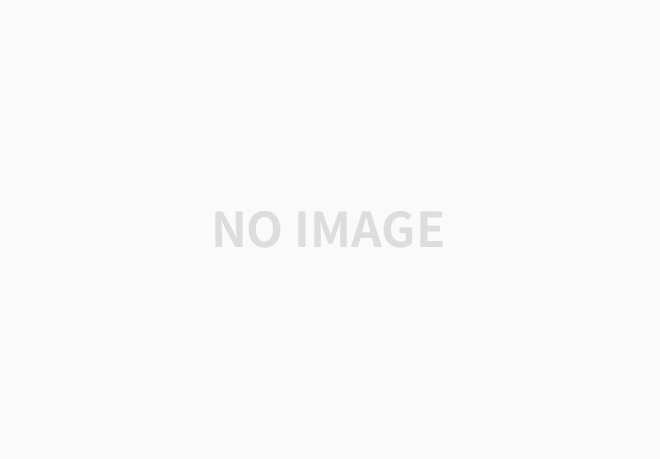
JPA와 H2에 대한 기본적인 기능과 설정을 진행했으니, 본격적으로 API를 만들어보자.
728x90
'기록 > 스프링 부트와 AWS로 혼자 구현하는 웹 서비스' 카테고리의 다른 글
Chapter 3. 프로젝트에 Spring Data JPA 적용하기 (0) | 2022.10.08 |
---|---|
Chapter 3. 스프링 부트에서 JPA로 데이터베이스 다뤄보기 (0) | 2022.10.07 |
Chapter 2. Hello Controller 코드를 롬복으로 전환하기 (0) | 2022.10.04 |
Chapter 2. 스프링 부트에서 테스트 코드 작성 (0) | 2022.09.29 |
Chapter 2 - 롬복 소개 및 설치하기 (0) | 2022.09.24 |